Developing an application that sends emails is straightforward but not without its risks. Ensuring deliverability but not actually having any of those emails land inside real inboxes is a top concern for any developer. Which leads to questions like: “How do you test your application’s outbound email capabilities?” or “How do I manage email testing for free?”
Enter email capture services. While the term “email capture service” tends to focus on the marketing aspects (capturing information from your calls to action, ensuring emails don’t get caught in spam, etc) they also include SMTP deliverability. Mailsac offers an email capture service that addresses the deliverability aspect, specifically not delivering any email to its intended recipient. Effectively a “black hole” where no email should escape to the outside world.
In this post, we’ll walk through a sample application in Next.js that will generate emails and have those emails captured by Mailsac’s email sandboxing service.
Do I Really Need To Do Email Testing?
Some frameworks do come with email previewing capabilities like Rails’ ActionMailer. Said frameworks don’t actually attempt to send anything but instead preview the email on your machine. We recommend real testing during the development and quality assurance phase by using an external SMTP server to mimic the application’s behavior in production.
Testing that capability has to be done safely unless you want to land on Twitter’s trending page for accidentally sending customers an integration test email.
Test Email Sending With A Next.js Application
For the rest of this guide, we’ll focus on wiring up a simple application that will allow users to send an email when a button is pushed from a UI. We’ll then demonstrate the capture of those emails in our development environment.
The components we’ll use are:
- Next.js with a React frontend and API Routes backend
- nodemailer package for routing email on the backend
- Mailsac’s Email Capture service as our SMTP service
Application Creation
While the focus of this guide isn’t a line-by-line walkthrough of the sample code, we’ll focus on the key aspects of the application that mainly involve emailing capabilities.
The application source can be found in our git repository.
1. Application setup
Let’s start by creating a quick next app with tailwind support:
mailsac % npx create-next-app
…
Success! Created nodejs-send-email at /Users/mailsac/code/nodejs-send-email
cd nodejs-send-email
npm install -D tailwindcss postcss autoprefixer @tailwindcss/forms
npm install @headlessui/react@latest @heroicons/react
npx tailwindcss init -p
The above is the recommended way to install tailwind on Next.js according to their guide.
Configure tailwind.config.js by adding the highlighted lines:
module.exports = {
content: [
"./pages/**/*.{js,ts,jsx,tsx}",
"./components/**/*.{js,ts,jsx,tsx}",
],
theme: {
extend: {},
},
plugins: [
require('@tailwindcss/forms'),
],
}
and then add tailwind itself to the global CSS file inside styles/global.css
and comment out some default CSS created by npx:
/* @media (prefers-color-scheme: dark) {
html {
color-scheme: dark;
}
body {
color: white;
background: black;
}
}
*/
@tailwind base;
@tailwind components;
@tailwind utilities;
Tailwind is strictly optional but recommended for easy styling of the frontend.
2. Add the front page UI
Feel free to add your custom frontend code or use the index.js
and components/notifications.js
react component samples inside our repo.
index.js
import { useEffect, useState } from "react";
import Notification from "../components/notifications";
export default function Index() {
const [sentEmail, setSentEmail] = useState(false);
const [emailTo, setEmailTo] = useState("");
const [emailBody,setEmailBody] = useState("");
const [resultMessage, setResultMessage] = useState("");
const sendEmail = () => {
setSentEmail(true);
}
useEffect( () => {
fetch('/api/send-email',{
method: 'POST',
body: JSON.stringify({ to: emailTo, body: emailBody})
})
.then( res => res.json())
.then(response => {
setResultMessage(response.message)
})
.catch(error => console.log(error));
},[sentEmail]);
const SentEmailBanner = sentEmail === true? <Notification message={resultMessage} /> : null;
The second line brings in a component that takes in a message and formats it as a pop-up notification. The useEffect()
method sends your email recipient and body input to the backend, which will forward that data to Mailsac’s servers.
3. Sign up for Mailsac’s Email Capture
Mailsac has a free email capture service. All you need is to sign up for an account and generate a key:

4. Add a backend mail handler route
Once you’ve generated and saved your keys, you can place them in a .env
file:
.env
MAILSAC_USERNAME=lcanal
MAILSAC_API_KEY=Key generated from above
Following our email capture documentation, we’ll create a backend API route for Next to handle the request:
pages/api/send-email.js
const nodemailer = require("nodemailer");
export default async function handler(req, res) {
let emailEnvelope = JSON.parse(req.body)
if (
req.method === 'POST'
&& typeof(emailEnvelope.to) !== 'undefined'
&& emailEnvelope.to !== ''
){
const mailsaUserName = process.env.MAILSAC_USERNAME
const mailsacAPIKey = process.env.MAILSAC_API_KEY
const transporter = nodemailer.createTransport({
host: 'capture.mailsac.com',
port: 5587,
// will use TLS by upgrading later in the connection with STARTTLS
secure: false,
auth: {
user: mailsaUserName,
pass: mailsacAPIKey
}
})
try {
const results = await transporter.sendMail({
from: '"Sample App" [email protected]',
to: emailEnvelope.to,
subject: 'Sample App Send',
text: emailEnvelope.body
})
res.status(200).json(
{
message: "You should now see an email in Mailsac's capture service",
response: results.data
}
)
} catch (error){
console.log(`ERROR ${error}`)
res.status(500).json({ message: `${error.response}`, response: error })
}
} else {
return res.status(200).json({message: "No data"});
}
}
In the highlighted line, we’re ensuring the useEffect()
hook gets called with input data before we allow the rest of the function to continue. useEffect()
gets called a variety of times in the component lifecycle, and this check is to ensure it was initiated by an end user and not as part of the component mounting.
5. Test driving the app
Fire up the application via
npm run dev
Navigate to http://localhost:3000
and type a text message:
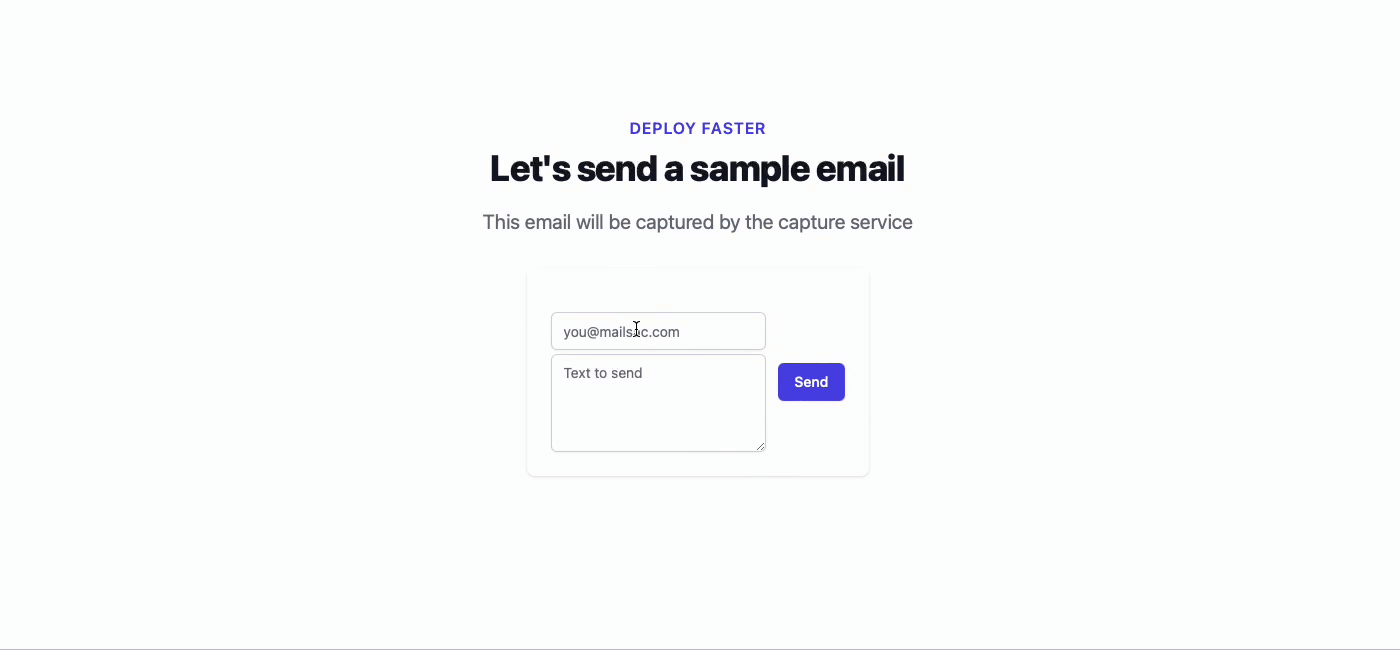
Then navigate over to mailsac.com to view the message
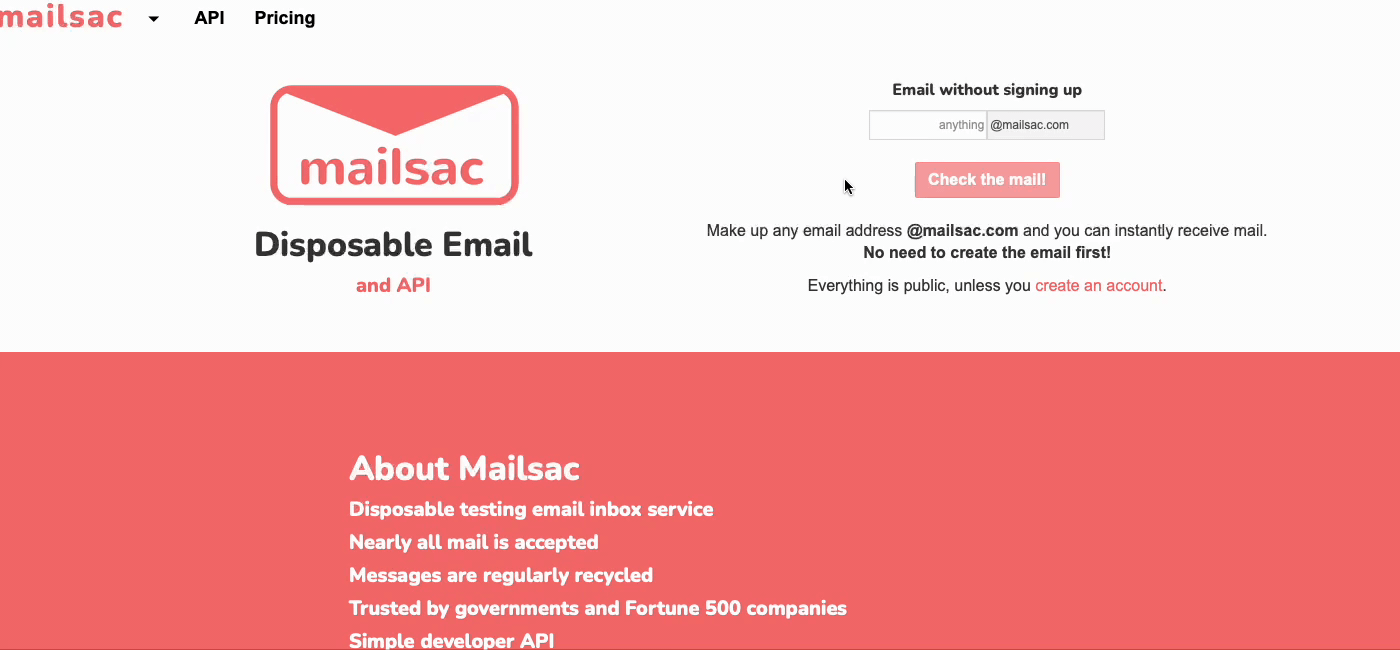
6. Capturing other email domains
While that works well as a contrived example, the real value comes when using any arbitrary email in the recipient field:
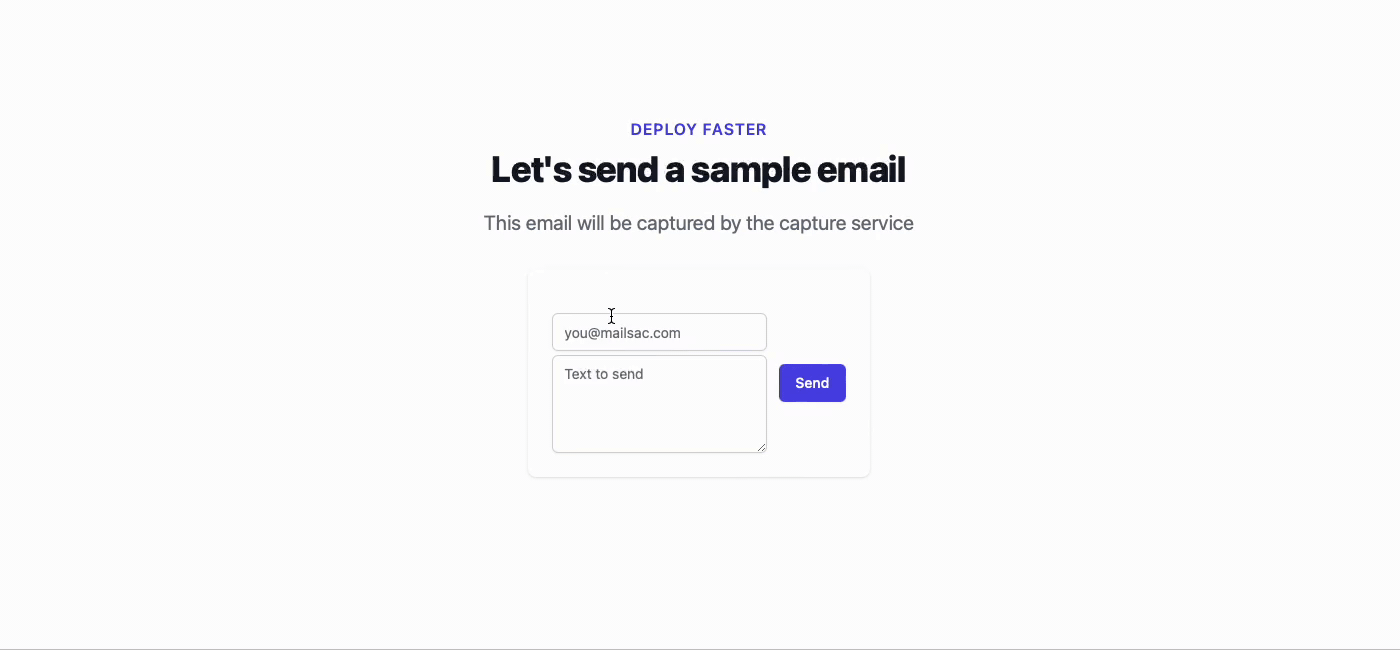
Capturing emails outside the mailsac.com domain is extremely valuable when switching between different environments. For example, in the demo application example above, the .env
environment file could instead look like
MAILSAC_USERNAME=$MAILSAC_NAME
MAILSAC_API_KEY=$MAILSAC_KEY
MAILSAC_HOST=capture.mailsac.com
MAILSAC_PORT=5587
With the updated send-email.js
const nodemailer = require("nodemailer");
export default async function handler(req, res) {
let emailEnvelope = JSON.parse(req.body)
if (
req.method === 'POST'
&& typeof(emailEnvelope.to) !== 'undefined'
&& emailEnvelope.to !== ''
){
const mailsaUserName = process.env.MAILSAC_USERNAME
const mailsacAPIKey = process.env.MAILSAC_API_KEY
const transporter = nodemailer.createTransport({
host: process.env.MAILSAC_HOST,
port: process.env.MAILSAC_PORT,
// will use TLS by upgrading later in the connection with STARTTLS
secure: false,
auth: {
user: mailsaUserName,
pass: mailsacAPIKey
}
})
try {
const results = await transporter.sendMail({
from: '"Sample App" [email protected]',
to: emailEnvelope.to,
subject: 'Sample App Send',
text: emailEnvelope.body
})
res.status(200).json(
{
message: "You should now see an email in Mailsac's capture service",
response: results.data
}
)
} catch (error){
res.status(500).json({ message: `${error.response}`, response: error })
}
} else {
return res.status(200).json({message: "No data"});
}
}
The above edits would allow you to deploy to a testing or production environment and the only changes required would be in the .env file. Specifically, the SMTP host and authentication settings.
Conclusion
The above guide just scratches the surface of what you can do with our email services. We provide a unified inbox that allows testers to view their bulk email testing in one unified view and custom domains for those who do not have their own domains with zero setup configurations.
Additionally, we have a guide on using both custom domains and a unified inbox in your system testing. Check it out and tell us what you think on our forums!