Developing and testing an application is difficult enough without the stress of GDPR, CAN-SPAM, accidental information leak, etc. But it doesn’t have to be.
In this article I’ll walk you through how our email platform could’ve helped prevent Running Warehouse from exposing thousands of their customer’s emails.
Background on the Running Warehouse Incident
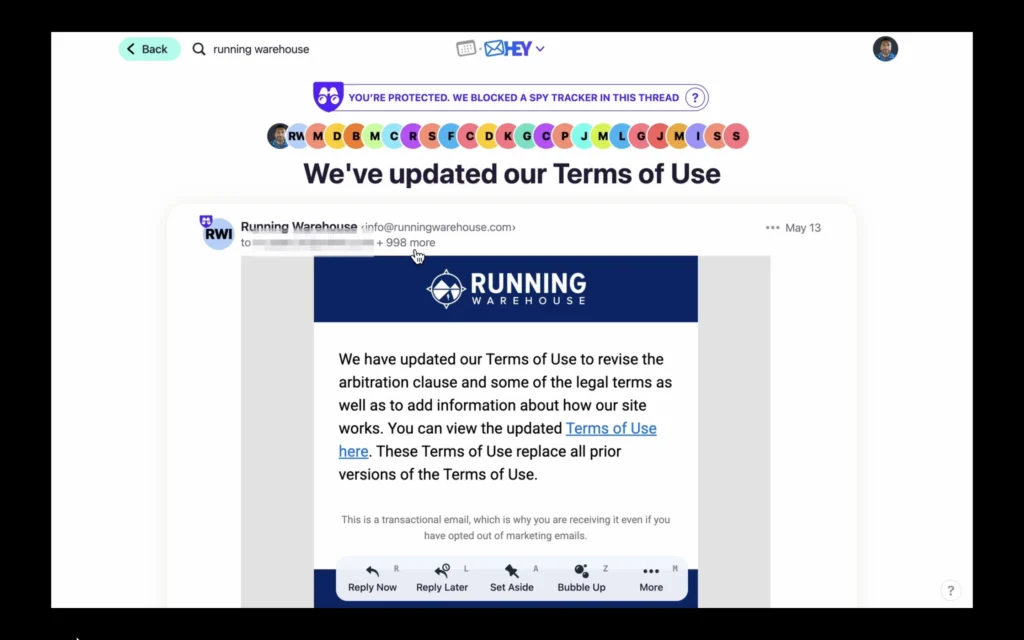
Now, this isn’t meant to poke fun at a company’s mistake but really to highlight just how easy these mistakes are to miss. Last month, I received two emails from “Running Warehouse” about an update to their terms of service. Nothing really unusual at first glance… Until you notice that about 1000 other customer’s email addresses had been sent along in the CC field. And to add even more insult to injury, they did this TWICE. So now I have a list of about 2000 of Running Warehouse’s customers that I’m sure the customers themselves are not happy about.
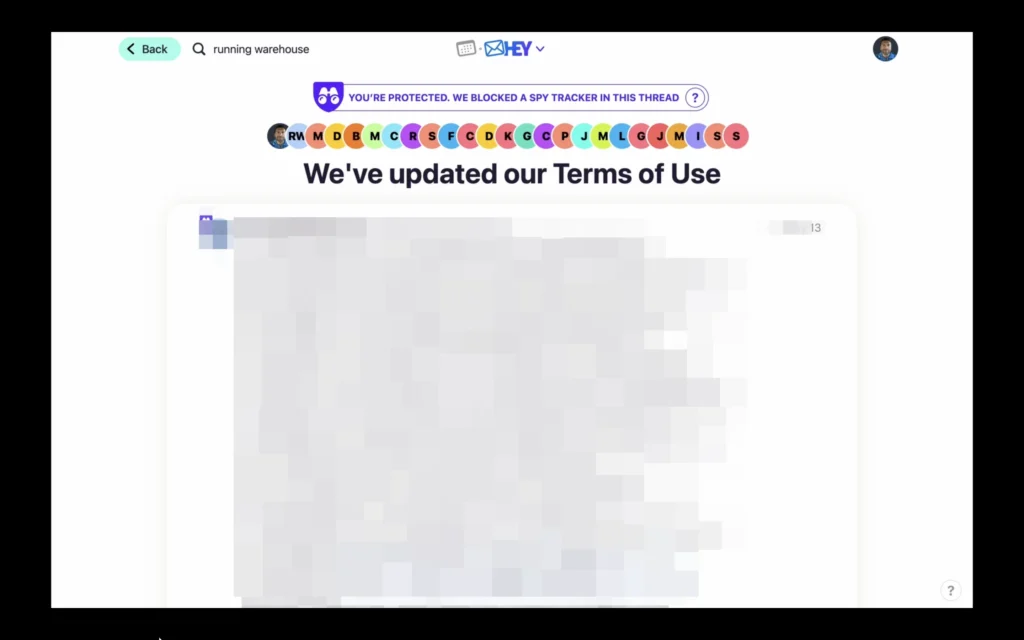
Really not a good look for a company who could be facing a class action lawsuit for a previous data breach back in 2021.
So let’s look at the implications of this easy to do mistake:
- Potential GDPR violations
- Loss of customer trust
- Company reputation lost via social media, youtube videos about you, forums, etc.
Prevent these errors with Mailsac
Obviously no company wants that! This is where mailsac steps in. We help prevent these kinds of easy mistakes, but at the application level. We have a set of features where you can hook up your continuous integration with our API to ensure the email you want to send is the one the recipient actually received.
So let’s walk through how we’d prevent this if this email was coming from our application.
Technical Deep-Dive
Here’s what we’ll do:
- Setup a sample application that is meant to simulate sending emails.
- Setup our Mailsac API Key.
- Send a sample email.
- Use the API to ensure our cc and email fields match what we sent.
Let’s get started.
Sample Application
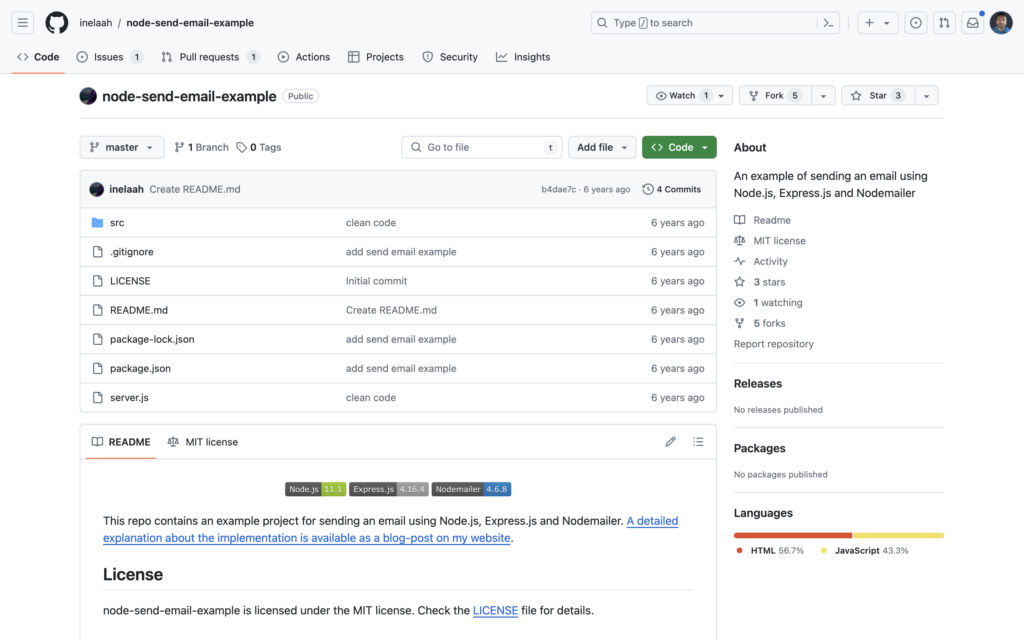
- To stand in place of your application, we’ll use this dead simple node app whose sole purpose is to just send an email based on what you place in a form.
- To simulate our email service API key, we’ll use our Application Key we generated for our gmail account. You can do this too if you want to step through this part. You can find it under your Google account -> security -> 2 FA -> App Password or just search for app passwords.
- Add your credentials in the config.js file directly.
- Again, while we embed the code here, you should really use a dot-env library.
- Most importantly, for our example here, let’s use a mailsac temporary address. We have ad hoc inbox creation abilities so for now, let’s say we’ll send it to [email protected]
- Fire up the app
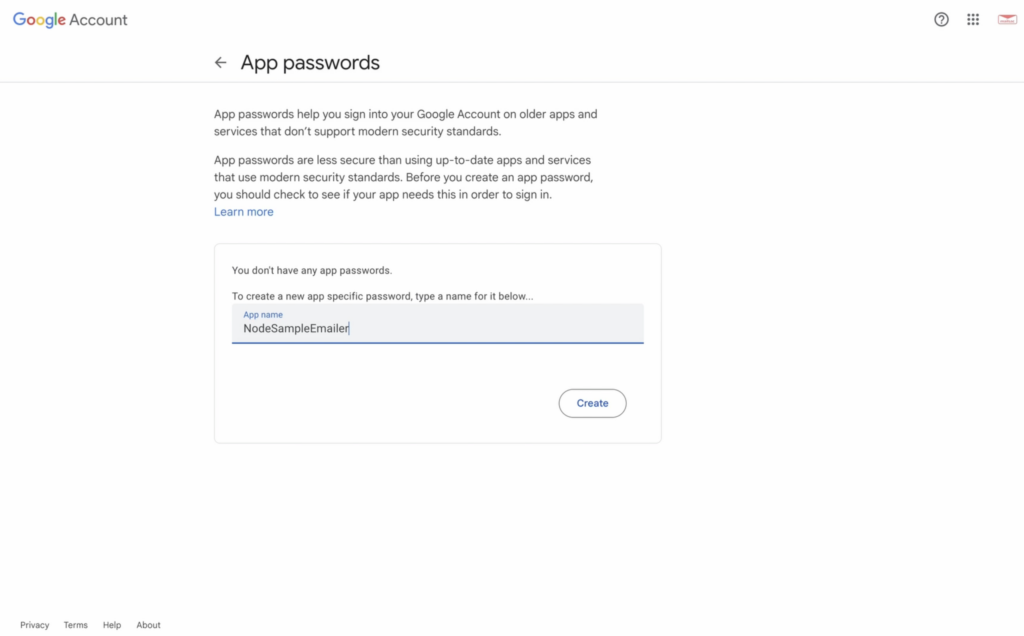
Setup our Mailsac API Key
Before we fire off an email, let’s add some quick code to check whether what we send is the same or not once it’s received.
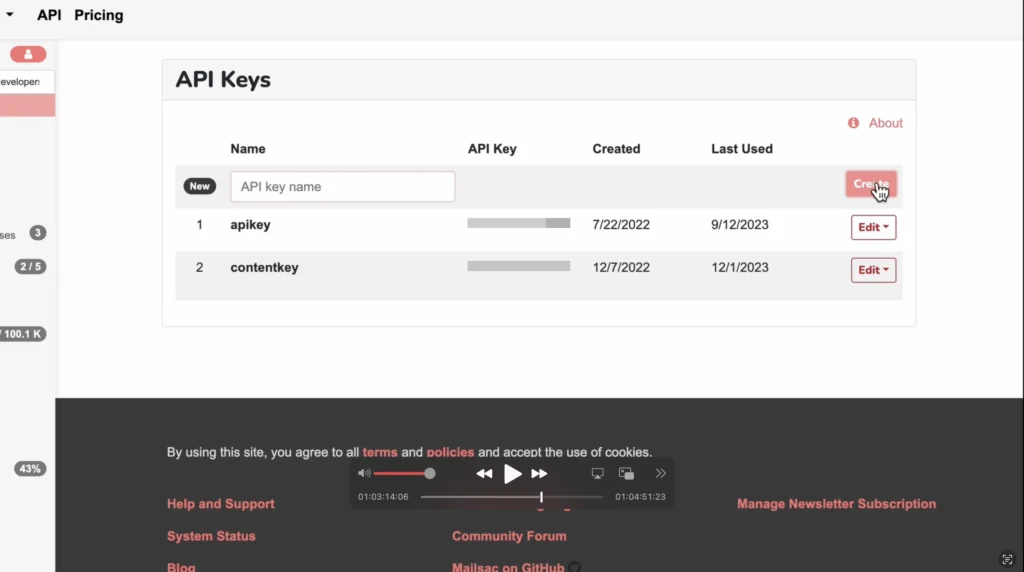
- Head to your Mailsac dashboard
- Generate a new key if you don’t have one
- Grab our api library (
npm install @mailsac/api
) - Write a test checker after a 1 second wait
The Email CC Checker Snippet
await setTimeout( async () => {
const mailsac = new Mailsac({ headers: { "Mailsac-Key": process.env.MAILSAC_API } })
const results = await mailsac.messages.listMessages("[email protected]")
const messages = results.data;
if (messages[0].cc.length <= 0){
console.error("This email has no CC. That's Good!")
}
if (messages[0].subject === req.body.subject){
console.error("This has the correct subject. That's Good!")
}
}, 1000);
Normally we’d place it in an .env file, but for our purposes here, we’ll just place it directly in the headers.
Send Sample Email
Alright now let’s fire this up and fire off an email
- Let’s make the content and subject something specific like:
- Subject:
This email is intended only for emailcontentcheck
- Content:
Hey, this email is strictly for [email protected]. No others on cc field.
- Subject:
- Fire it off
- Wait for the check to fire off and print out comparison / success
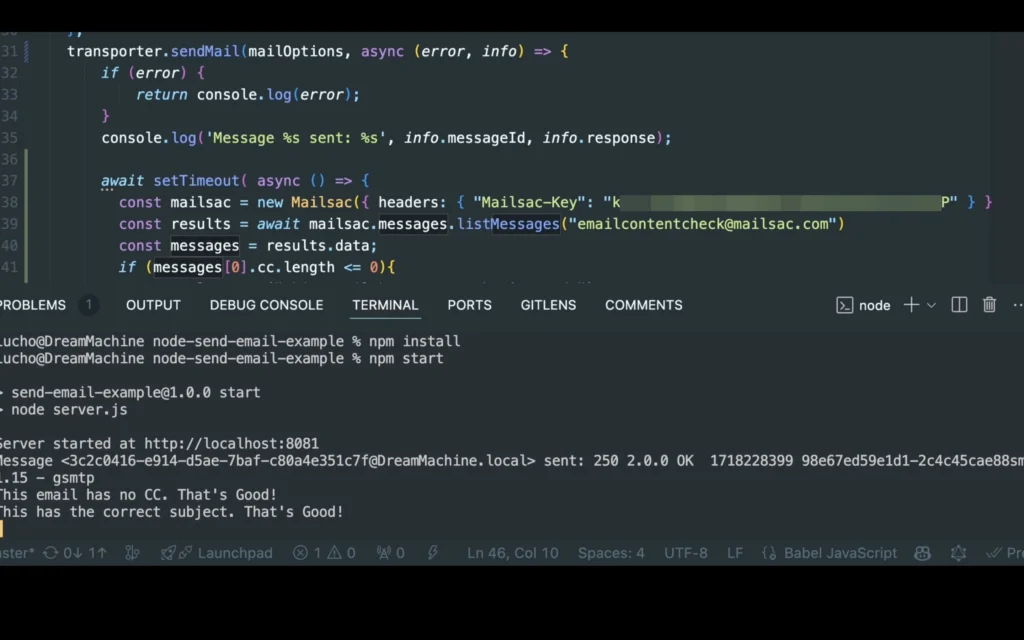
Use the API to ensure our cc and email fields match what we sent
Right after our code fires off, it should print out the message comparison result. But now, let’s make sure our CC fields are also empty
await setTimeout( async () => {
const mailsac = new Mailsac({ headers: { "Mailsac-Key": process.env.MAILSAC_KEY } })
const results = await mailsac.messages.listMessages("[email protected]")
const messages = results.data;
if (messages[0].cc.length <= 0){
console.error("This email has no CC. That's Good!")
}
if (messages[0].subject === req.body.subject){
console.error("This has the correct subject. That's Good!")
}
}, 1000);
We’re even throwing in a subject line check. You can check out the full API documentation to see how you can check for empty BCCs, content checks, and more.
Fire it off again and enjoy having an automated way test your email content, cc fields, and more!
Wrap Up
Finding the right words to use in an email is already hard enough, don’t complicate it by worrying about whether the email went through, if it works right, if it accidentally cc’s people, etc.
Just let mailsac handle the double checking for you.
And if you want to dive even deeper into email testing, we have a full set of articles on integrating with Cypress or integrating other testing tools like Selenium with GitHub Actions for a fully automated testing pipeline.